Multi-Tier Architecture for Healthcare Data Processing with Performance Guarantees
As a continuation of previous article
Healthcare information systems encounter unique challenges when processing time-sensitive clinical data. While standard cloud architectures satisfy general-purpose workloads, they often fall short when handling the mixed-priority data streams typical in healthcare environments. This article explores an architectural approach that addresses these performance issues without excessive resource provisioning.
Mixed-Priority Data in Healthcare
Healthcare systems process a wide spectrum of data types with significantly different performance requirements:
- Critical diagnostic results that affect immediate clinical decisions
- Real-time patient monitoring data from ICU and surgical environments
- Standard clinical documentation with moderate time sensitivity
- Bulk administrative data and analytics workloads
Standard cloud infrastructure typically processes these diverse data types through identical pathways with uniform retry logic. When system load increases, this architectural approach can cause condition when critical information experiencing the same delays as routine data.
This approach of relying on excessive resource provisioning leads to significant cost inefficiencies without resolving the core architectural challenge.
Standard Cloud Architecture Limitations
The default approach in cloud environments relies heavily on retry patterns to handle processing failures:
func processHealthcareData(ctx context.Context, data []byte) error {
// Standard exponential backoff retry policy
backoff := retry.NewExponentialBackOff()
backoff.InitialInterval = 100 * time.Millisecond
backoff.MaxInterval = 10 * time.Second
backoff.MaxElapsedTime = 1 * time.Minute
err := retry.Do(
func() error {
return processingService.Process(ctx, data)
},
retry.WithContext(ctx),
retry.WithBackoff(backoff),
)
return err
}
While this approach ensures data eventually gets processed, it provides no mechanism for distinguishing between a routine administrative record and a critical lab result that could impact immediate patient care. During system load spikes, critical information can experience unpredictable delays.
An alternative architectural approach is needed that specifically addresses the mixed-priority nature of healthcare data and provides differentiated processing paths based on clinical urgency.
Architectural Comparison
The fundamental difference between standard and priority-based approaches lies in how they route and process data of varying clinical importance:
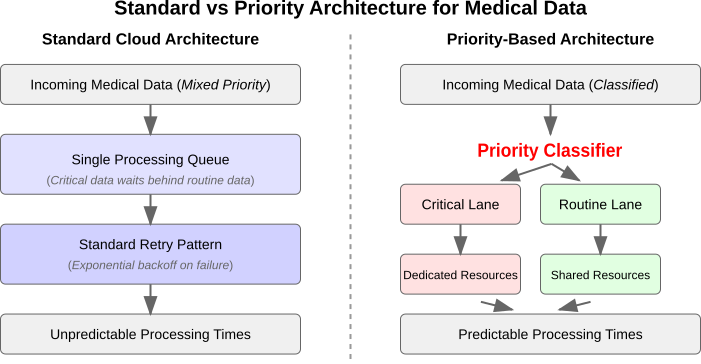
Figure 1: Standard vs Priority-Based Architecture
Standard architecture (left) processes all data through a single queue with uniform retry logic, while the priority-based approach (right) implements separate processing paths with dedicated resources for data of different criticality levels. This separation is the key to providing performance guarantees for time-sensitive clinical information.
Multi-Tier Processing Architecture
Building on the priority-based concept shown above, a comprehensive multi-tier approach implements dedicated processing lanes with separate resource allocations based on data priority:
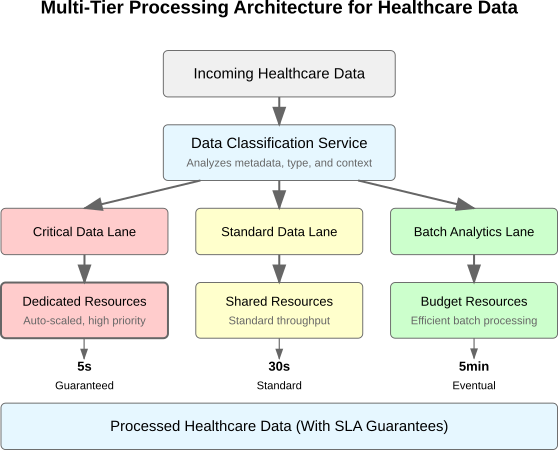
Figure 2: Multi-Tier Architecture for Healthcare Data Processing
This architecture separates incoming healthcare data into distinct processing lanes:
- Critical Lane: For time-sensitive clinical data requiring immediate processing
- Standard Lane: For routine clinical information
- Batch Lane: For analytics, billing, and administrative data
Each lane maintains dedicated processing resources, ensuring that load spikes in lower-priority queues cannot impact the performance of critical data pathways.
Implementation: Priority-Based Workload Scheduler
The core of this architecture is a priority-based workload scheduler that routes incoming data to appropriate processing lanes while maintaining data integrity:
type DataPriority int
const (
Critical DataPriority = iota
Standard
Batch
)
type HealthcareData struct {
Priority DataPriority
Payload []byte
Metadata map[string]string
}
// WorkloadScheduler routes healthcare data to appropriate processing lanes
type WorkloadScheduler struct {
criticalQueue chan HealthcareData
standardQueue chan HealthcareData
batchQueue chan HealthcareData
criticalPool *worker.Pool
standardPool *worker.Pool
batchPool *worker.Pool
}
func (s *WorkloadScheduler) Schedule(ctx context.Context, data HealthcareData) error {
switch data.Priority {
case Critical:
// Use context with shorter timeout for critical data
ctxCritical, cancel := context.WithTimeout(ctx, criticalTimeout)
defer cancel()
select {
case s.criticalQueue <- data:
return nil
case <-ctxCritical.Done():
// If critical queue is full, handle as escalation
return s.handleCriticalOverflow(ctx, data)
}
case Standard:
// Use context with standard timeout
ctxStandard, cancel := context.WithTimeout(ctx, standardTimeout)
defer cancel()
select {
case s.standardQueue <- data:
return nil
case <-ctxStandard.Done():
// If standard queue times out, attempt to requeue
return s.handleStandardOverflow(ctx, data)
}
case Batch:
// For batch data, use non-blocking send with fallback
select {
case s.batchQueue <- data:
return nil
default:
// Store in overflow buffer for later processing
return s.storeBatchData(ctx, data)
}
}
return nil
}
This implementation demonstrates how the scheduler routes each incoming data item to the appropriate processing lane based on its priority classification. Unlike the standard approach shown earlier, this pattern provides explicit handling for each priority level with different context timeouts, queue management strategies, and escalation paths.
Resource Allocation Strategy
Optimizing resource allocation represents a key challenge in implementing multi-tier processing architecture. The system must balance performance guarantees against infrastructure costs.
An effective approach to resource utilization requires finding a balance point between:
- Sufficient headroom for traffic spikes (avoiding performance degradation)
- Efficient resource utilization (avoiding unnecessary costs)
- Reliable performance under varying workloads
This balance applies differently to each processing lane:
- Critical Lane: Higher resource allocation with increased headroom to ensure immediate capacity
- Standard Lane: Moderate resources optimized for consistent performance
- Batch Lane: More conservative resources as latency is less critical for these workloads
The key insight is that different data types require different resource allocation strategies based on their clinical importance and operational requirements. This contrasts with the one-size-fits-all approach of traditional cloud architectures.
Implementation Challenges
Organizations implementing multi-tier architecture should anticipate several challenges:
- Data Classification Logic: Developing robust algorithms to correctly classify incoming data by priority
- Resource Allocation Balance: Finding the optimal provisioning for each tier based on workload patterns
- Monitoring Complexity: Implementing distinct performance monitoring for each processing lane
- Cross-Lane Integrity: Ensuring data integrity when related information flows through different lanes
Addressing these challenges requires both technical expertise and domain knowledge of healthcare workflows. The classification logic, in particular, needs to incorporate clinical urgency factors rather than purely technical metrics.
Conclusion
Multi-tier processing architecture provides healthcare organizations with a systematic approach to guaranteeing performance for time-sensitive clinical data. By implementing dedicated processing lanes with appropriate resource allocation, organizations can ensure critical information reaches clinicians when needed without excessive infrastructure costs.
The key benefit extends beyond technical performance metrics to tangible clinical outcomes—ensuring that life-critical information flows through the system with appropriate priority and guaranteed response times.
Further Reading
Academic Perspective:
A priority queue-based telemonitoring system for automatic diagnosis of heart diseases in integrated
fog computing environments
Healthcare Implementation:
Priority Queues in Healthcare: Optimizing Patient Care
Technical Background:
Application of Priority Queue in Real-Life (Explained)
Cloud Design Patterns:
Retry Pattern in Cloud Design Patterns documentation